Sending Laravel Production App Logs to Better Stack
Published Feb 13, 2024I’m using a managed hosting service that uses an ephemeral multi node environment. They’ve been great, but they don’t provide historical logging. Which is a problem. I need this information in case anything goes wrong in production. Ideally, I use the logs to catch something before it turns into a real problem.
If you look in the Laravel documentation, you’ll find a couple third-party channels listed. One of those is Papertrail, which provides pretty decent looking pricing and a generous no credit card free trial. You only get 50mb per month for your log storage though. That might be okay, but I opted for a similar service that allows 1gb per month instead. That service is called Better Stack.
This is how you set it up.
Sign up for a no credit card free account on Better Stack. Once you’re logged in, click on “Connect source”.
Give your source a name and select PHP as your platform. Click “Create source”.
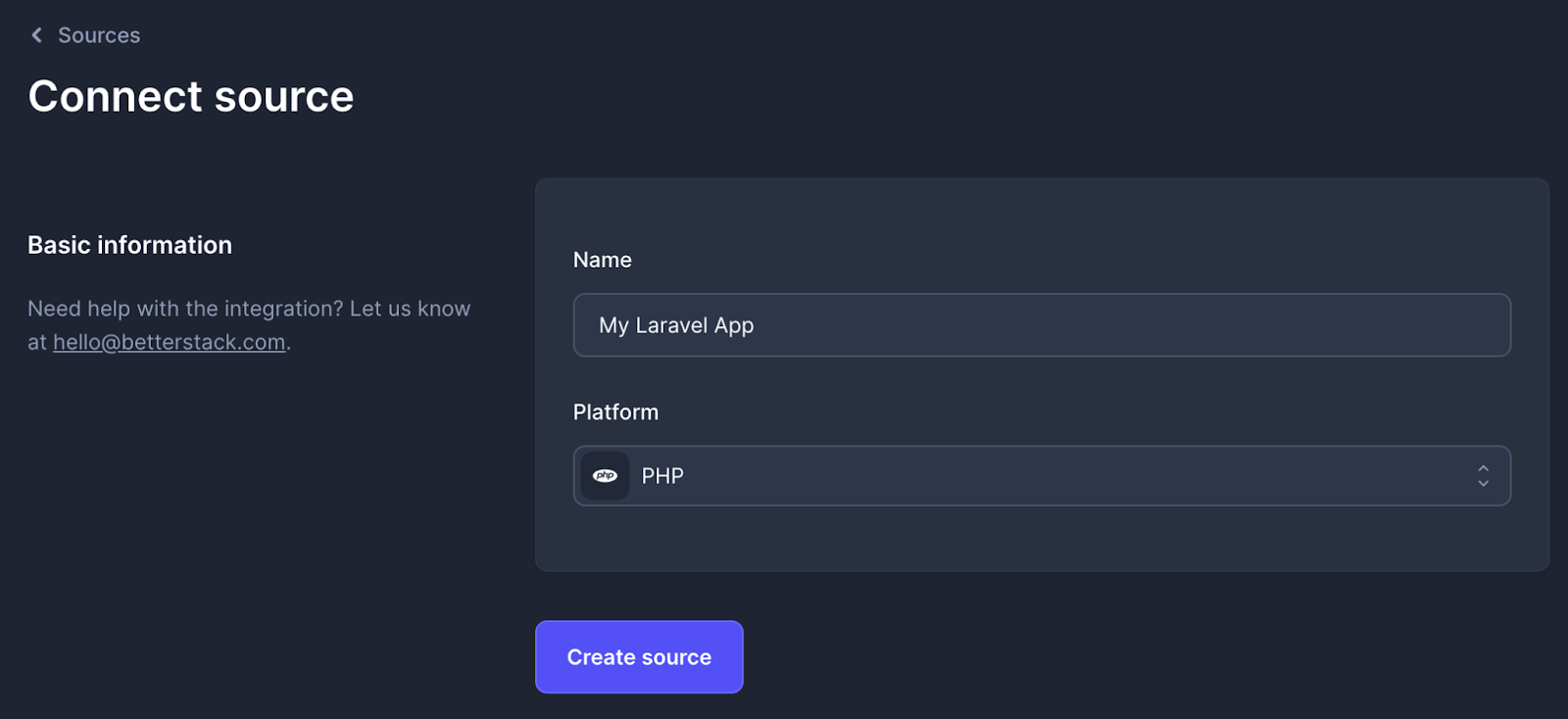
From here, you’ll be given a source token. Click on it to copy it.
In your .env
file, add that source token and change your LOG_CHANNEL
like this.
BETTERSTACK_SOURCE_TOKEN="AAAAAAAAAAAAAAAAAAAA"
LOG_CHANNEL=betterstack
But that channel doesn’t actually exist yet. We’ll create it next, but first let’s install a package that will make that easier. Better Stack used to be called LogTail. Install the logtail package using composer.
composer require logtail/monolog-logtail
Then run composer dump-autoload
so that composer refreshes your classmap and the new package’s classes can be found.
Now, within your config/logging.php
file, add our new channel to the channels
array.
'betterstack' => [
'driver' => 'monolog',
'level' => env('LOG_LEVEL', 'debug'),
'handler' => Logtail\Monolog\LogtailHandler::class,
'handler_with' => [
'sourceToken' => env('BETTERSTACK_SOURCE_TOKEN'),
],
],
That’s it! You should now be able to tail your logs in Better Stack.
Test it out real quick by adding a log route to your app’s web.php
file.
Route::get('/log', function() {
\Illuminate\Support\Facades\Log::info("Hello BetterStack!");
return “log sent”;
});
Hit that in your browser. If you get a 404, then clear your route cache with php artisan route:cache
.
Now check the Live tail in Better Stack. You should get the log.
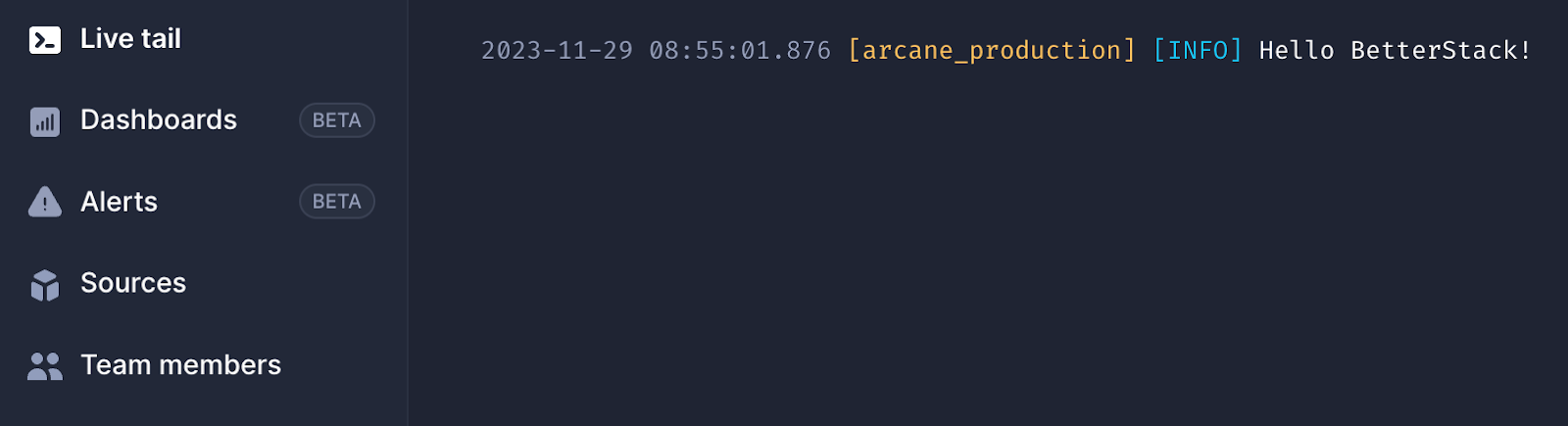
Okay! So that will stick around for 3 days. Say you want to archive it. You can hook it up to an S3 bucket, but it’s a little tricky to find after you’ve already created the bucket. I found a link to the S3 Bucket integration in their documentation.
Add your secret key and access key for AWS to your .env file.
Create a private S3 bucket in AWS and give it a bucket policy that looks like this.
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "Statement1",
"Effect": "Allow",
"Principal": {
"AWS": "the arn of the user that you used to give your app access"
},
"Action": [
"s3:PutObject",
"s3:GetObject",
"s3:ListBucket"
],
"Resource": [
"arn:aws:s3:::your-bucket-name",
"arn:aws:s3:::your-bucket-name/*"
]
}
]
}
Then fill out the AWS S3 integration form. Better Stack will test your connection. And you’re all set.